matplotlib基本用法
matplotlib用法
准备
1 |
|
基本图像绘制
折线图(Line Plot):
- 适用特征:用于显示数据随时间或有序类别变化的趋势,例如时间序列数据、股票价格等。
1 |
|
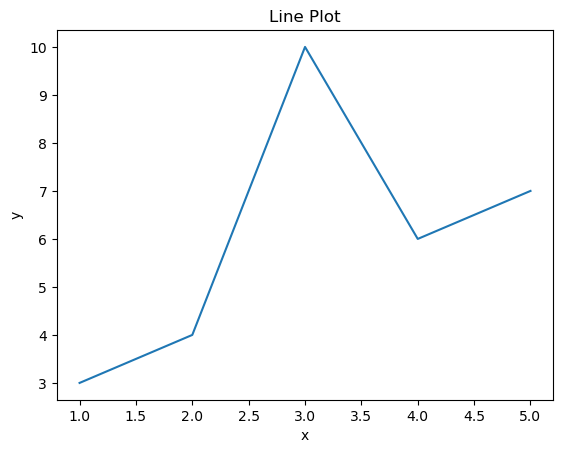
当然可以传入一些自定义的参数,看一组示例:
1 |
|
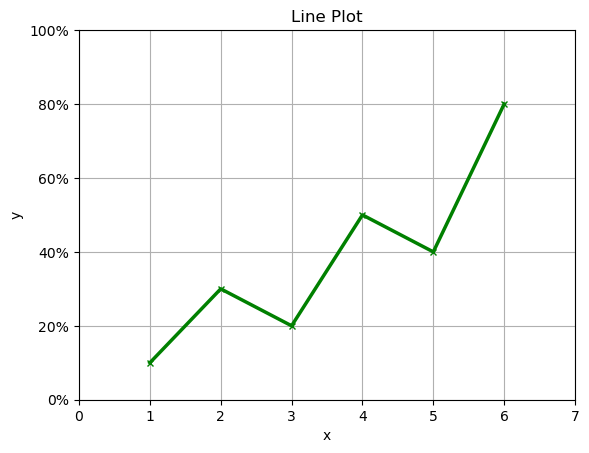
也可以包含多组折线
1 |
|
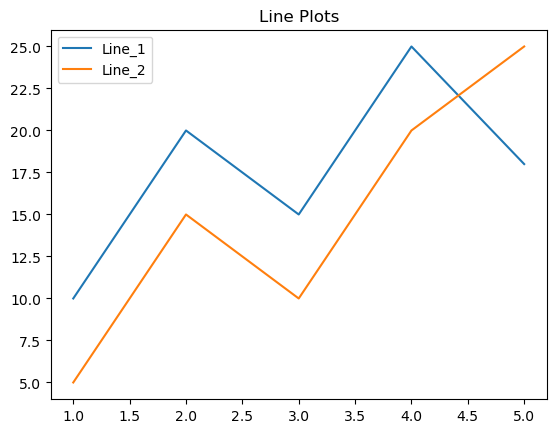
散点图(Scatter Plot):
- 适用特征:用于显示两个变量之间的关系,例如相关性、聚类等。
1 |
|
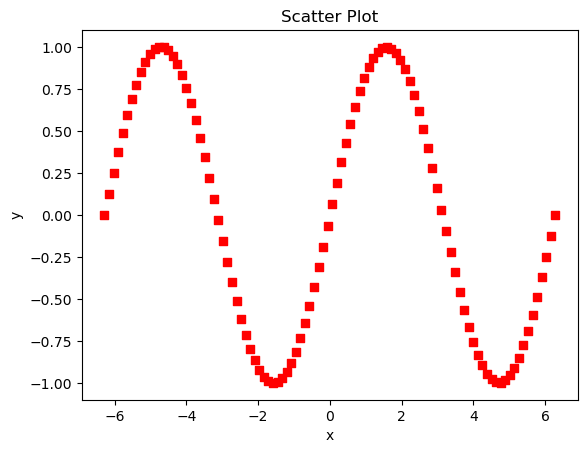
柱状图(Bar Plot):
- 适用特征:用于比较不同类别之间的数据,例如不同产品的销售额、不同地区的人口数量等。
1 |
|

当块数较多时,可以通过不同颜色来区分,提供两种方案
自定义有限数目的颜色,通过颜色循环填充较多的块
1
2
3
4
5
6
7
8
9
10categories = np.array(['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T'])
values = np.array([10, 20, 15, 25, 8, 12, 18, 6, 9, 22, 16, 14, 11, 7, 13, 19, 17, 23, 21, 24])
colors = ['red', 'blue', 'green']
bar_colors = [colors[i % len(colors)] for i in range(len(categories))]
plt.bar(categories, values, color=bar_colors,alpha=0.3)
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')使用一种颜色,通过matplotlib的内置颜色映射,根据y_values的数值大小划分颜色深浅(推荐)
1
2
3
4
5
6
7
8categories = np.array(['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T'])
values = np.array([10, 20, 15, 25, 8, 12, 18, 6, 9, 22, 16, 14, 11, 7, 13, 19, 17, 23, 21, 24])
normalized_values = (values - np.min(values)) / (np.max(values) - np.min(values)) #归一化
plt.bar(categories, values, color=plt.cm.Greens(normalized_values))
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')在此基础上,可以给每个bar添加数值标签
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21categories = np.array(['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T'])
values = np.array([10, 20, 15, 25, 8, 12, 18, 6, 9, 22, 16, 14, 11, 7, 13, 19, 17, 23, 21, 24])
normalized_values = (values - np.min(values)) / (np.max(values) - np.min(values)) #归一化
plt.bar(categories, values, color=plt.cm.Greens(normalized_values))
plt.xlabel('Categories')
plt.ylabel('Values')
plt.title('Bar Plot')
for i, v in enumerate(values):
plt.text(categories[i], v, str(v), ha='center', va='bottom')
'''
plt.text用于打印文本,依次包含坐标参数,文本内容,水平对齐方式(ha),垂直对齐方式(va)
水平对齐方式:
`left`:将文本标签的左侧与给定坐标对齐。
`center`:将文本标签的中心与给定坐标对齐。
`right`:将文本标签的右侧与给定坐标对齐。
垂直对齐方式:
`top`:将文本标签的顶部与给定坐标对齐。
`center`:将文本标签的中心与给定坐标对齐。
`bottom`:将文本标签的底部与给定坐标对齐。
直方图(Histogram):
- 适用特征:用于显示数据的分布情况,例如连续变量的频率分布。
1 |
|
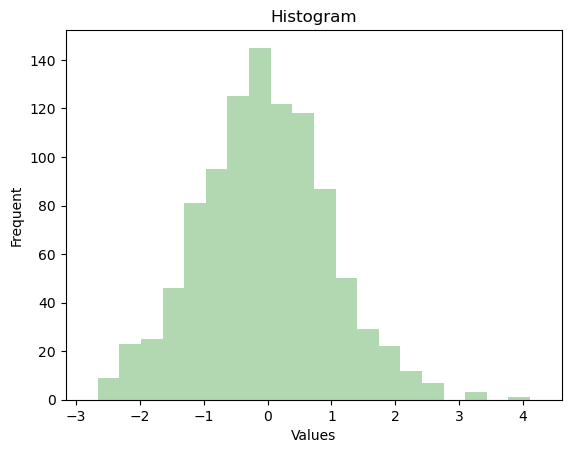
箱线图(Box Plot):
适用特征:用于显示数据的分布和异常值情况,例如数据的中位数、上下四分位数、离群值等。
1 |
|
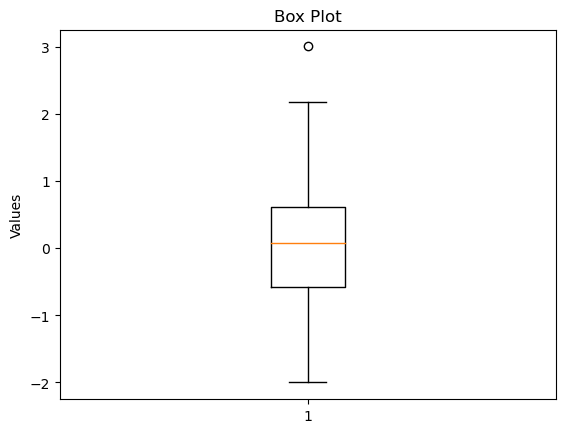
饼图(Pie Chart):
- 适用特征:用于显示不同类别在整体中的占比情况,例如不同产品的市场份额。
1 |
|
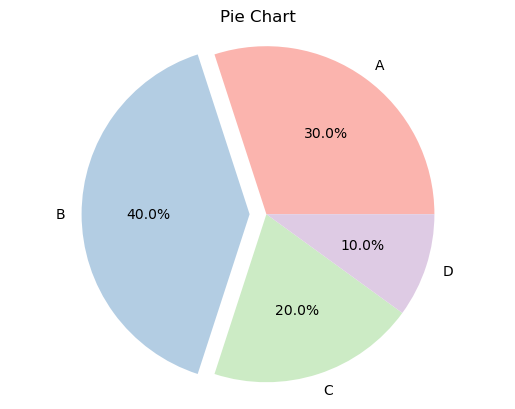
热力图(Heatmap):
- 适用特征:用于显示两个变量之间的关系,并通过颜色编码显示数值大小,例如相关矩阵、特征相关性等
1 |
|
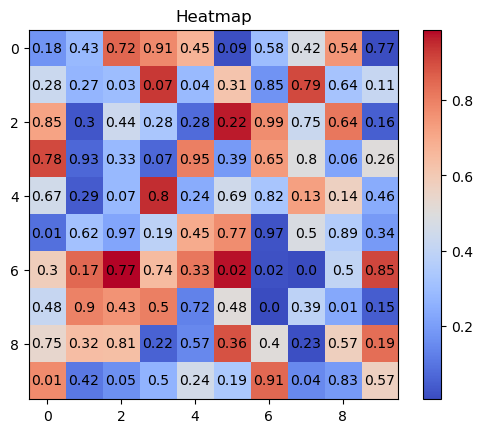
此种方法性能低下,理论上可以使用矩阵运算提高性能,未完待续……
matplotlib基本用法
https://blog.potential.icu/2023/12/29/matplotlib基本用法/